Python GUI: thinter 简单教程
tkinter 官方文档:
tkinter --- Tcl/Tk 的 Python 接口 — Python 3.12.8 文档
其他的一些文档:
Tkinter GUI 教程 – Tkinter 布局助手 (pytk.net)
Python GUI 之 tkinter 窗口视窗教程大集合(看这篇就够了) - 洪卫 - 博客园 (cnblogs.com)
有个很好的用来布局参考的工具,可视化、可拖拽:
最近项目需要我了解一下桌面端开发的一整套框架,基础框架中用到了 Python GUI 里的 thinter 包,所以简单做一些记录。
1. Tkinter 编程
tkinter 编程的主体框架:
1 |
|
2. 常用控件
基础组件
组件名称 | 组件说明 |
---|---|
Label | 标签 |
Entry | 输入框 |
Button | 按钮组件 |
Text | 文本框 |
Progressbar | 进度条 |
Radiobutton | 单选框 |
Checkbutton | 多选框 |
Listbox | 列表框 |
Combobox | 下拉选择框 |
Separator | 分割线 |
Spinbox | 数字输入框 |
OptionMenu | 下拉菜单 |
Menu | 菜单栏组件 |
Scale | 滑块组件 |
LabeledScale | 带有标签的滑块 |
BitmapImage | 图片组件 GIF |
Treeview | 树形组件、表格组件 |
Menubutton | 菜单按钮,点击按钮弹出菜单 |
Scrollbar | 滚动条 |
PhotoImage | 图片组件 PGM, PPM, GIF, PNG |
Canvas | 画布 |
Sizegrip | 尺寸调整组件 |
Frame 类组件
组件名称 | 组件说明 |
---|---|
Frame | 容器组件 |
LabelFrame | 标签框 |
PanedWindow | 分割面板 |
Notebook | 选项卡组件 |
3. tkinter 三种布局器
在 Tkinter 中,提供了三种布局方式。pack(打包)、grid(网格布局)、place(定位布局)。
布局方法 | 说明 |
---|---|
pack() | 按照组件添加到容器的顺序布局。在使用容器(Frame)布局时非常方便,调整窗口大小时布局自动缩放 |
grid() | 网格布局,以行、列来对组件进行布局,较为灵活 |
place() | 定位布局,指定组件大小和位置,最灵活 |
pack()
打包器,将组件打包进父组件中。
常用参数 | 说明 |
---|---|
anchor | 指定组件方向,取值 NSEW, 北南东西,和他们的组合方位,以及 Center |
expand | 如果父组件的大小增加,则展开当前组件 |
fill | 是否拉伸组件 NONE (不拉伸) 、X (横向拉伸)、Y (竖向拉伸)、BOTH (都拉伸) |
side | 将组件添加到哪 TOP、 BOTTOM 、 LEFT 、 RIGHT |
pack () 布局例子
1 |
|
pack 的布局跟添加的顺序有关,注意下面图的红色和蓝色相交位置,体会其中差别。
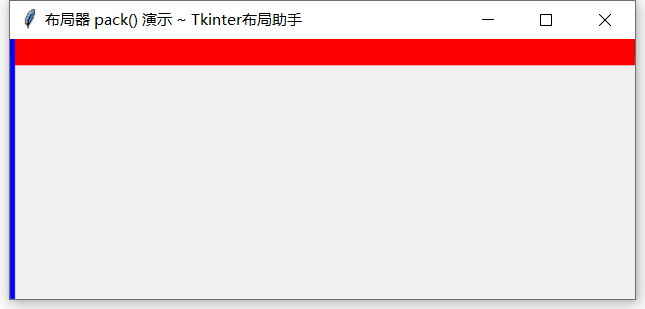
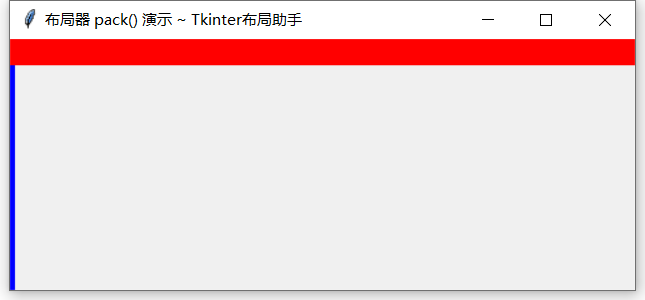
grid()
网格布局,用类似网格的形式,将界面分为几行,几列,每个组件布置在相应的格子里。
常用参数 | 说明 |
---|---|
column | 组件在第几列 默认从 0 开始 |
columnspan | 这个组件跨了几个列 |
row | 组件在第几行 默认从 0 开始 |
rowspan | 这个组件跨了几行 |
sticky | 组件在单元格内,靠近哪边,默认居中 |
grid () 布局例子
1 |
|
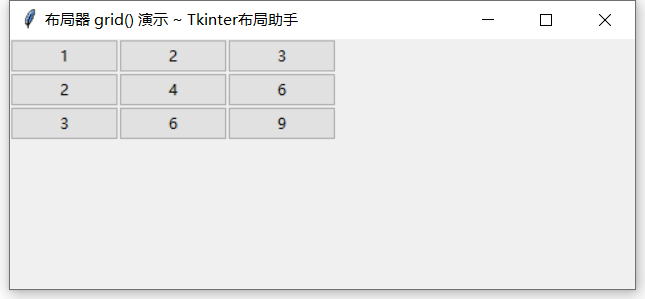
place()
三种中最灵活的一种,可以指定大小和绝对位置布局,也可以相对父组件的大小、相对位置布局。 Tkinter 布局助手就是基于 place () 的布局方式实现的。
常用参数 | 说明 |
---|---|
width | 组件的宽度 默认单位像素 |
height | 组件高度 默认单位像素 |
x | 相对父组件的 x 轴位置 (距顶部的距离) 默认单位像素 |
y | 相对父组件的 y 轴位置 (距左边的距离) 默认单位像素 |
relwidth | 组件的宽度 相对于父组件的宽度,取值 0-1 为 1 时与父组件宽度一致 |
relheight | 组件的高度 相对于父组件的高度,取值 0-1 为 1 时与父组件高度一致 |
relx | 相对父组件的 x 轴位置 (距顶部的距离) 取值 0-1 为 1 时与父组件宽度一致 |
rely | 相对父组件的 y 轴位置 (距左边边的距离) 取值 0-1 为 1 时与父组件高度一致 |
grid () 布局例子
1 |
|
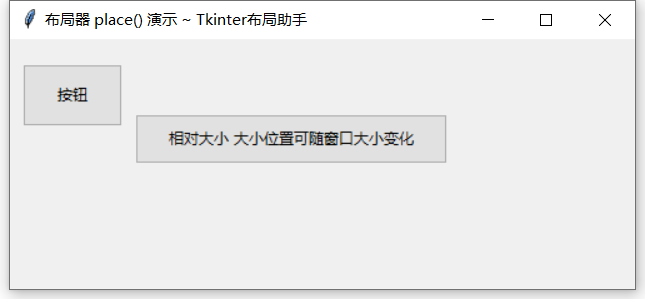
调整大小后,图中的小按钮大小和位置没有变化,设置相对大小的按钮大小发生了变化。
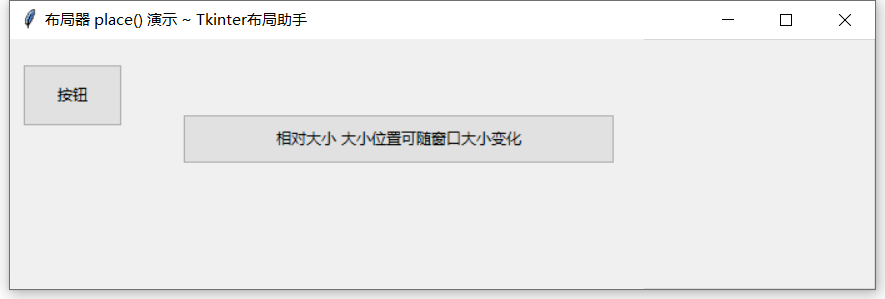
对此,那我们使用控件的时候,根据情况选择相对大小还是绝对大小,同样的,使用 tkinter 布局助手可以十分方便进行控件的布局。
4. 关于 TTK
Tkinter 模块是 Python 的标准 Tk GUI 工具包的接口。
Tk 和 Tkinter 可以在大多数的 Unix 平台下使用,同样可以应用在 Windows 和 Mac 系统里。Tk8.0 的后续版本可以通过 ttk 实现本地窗口风格,并良好地运行在绝大多数平台中。
尽量使用 ttk 组件。
TTK 效果演示
演示代码
1 |
|
截图
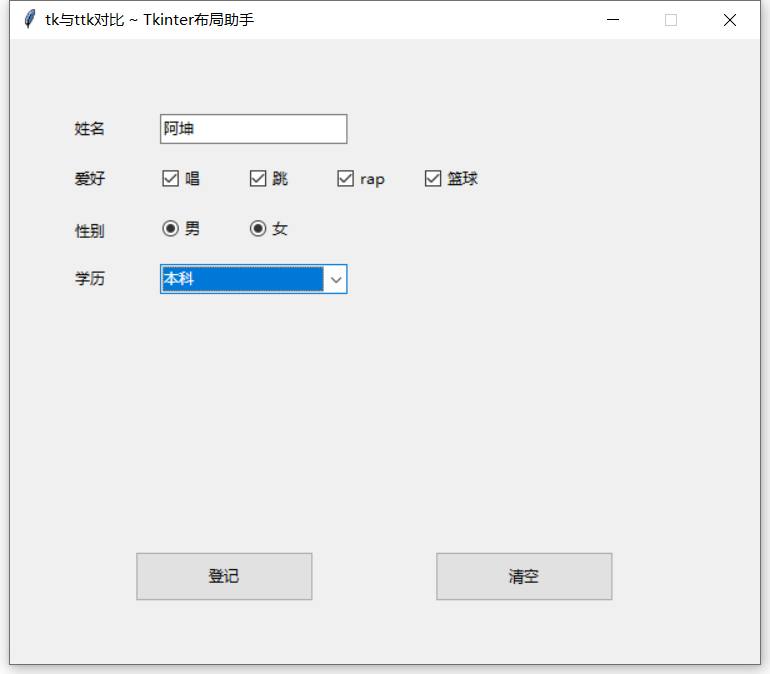
ttk 组件多于 tk,界面也相对 tk 漂亮,所以使用时尽量选择 ttk。因为 ttk 也是 python 原生支持的,不需要额外去下载。按照以下方式导入组件就行了,ttk 中的组件会默认替换掉 tk 的。
1 |
|
5. Label 标签
Label 标签组件,是最简单的组件之一。它是一个非交互式小部件,其唯一目的是向用户展示文字和图片。
常用属性
属性名 | 说明 |
---|---|
image | 指定要展示的图片。建议使用 PIL 的 Image, ImageTk 模块导入图片,少走弯路详见例子(PhotoImage 支持的格式较少,不支持图片缩放) |
compound | 混合模式。当 image 与 text 属性一起使用时。设置为 CENTER 文本则展示在图片上,其他选项包括 BOTTOM、LEFT、RIGHT、TOP 展示在图片旁边 |
cursor | 指定控件使用的鼠标光标 |
style | 设置样式 |
text | 要在标签中显示的文本字符串 |
textvariable | 指定一个变量,设置展示的文本,并在变量变化时,界面展示的文本自动更新 (类似前端 Vue 中的参数双向绑定) |
underline | 下划线。取值为数字,表示第几位字符开启下划线,取值从 0 开始,-1 为不开启下划线 |
width | 组件宽度 |
anchor | 控制内容在标签内的方向,默认居中。 |
background | 设置背景颜色 |
font | 设置字体 |
foreground | 前景色 |
justify | 文本的对齐方式。取值范围 left (左)、center (中)、right (右)。 |
padding | 组件内边距 (与 html 中的 padding 类似) |
Label 组件示例代码
1 |
|
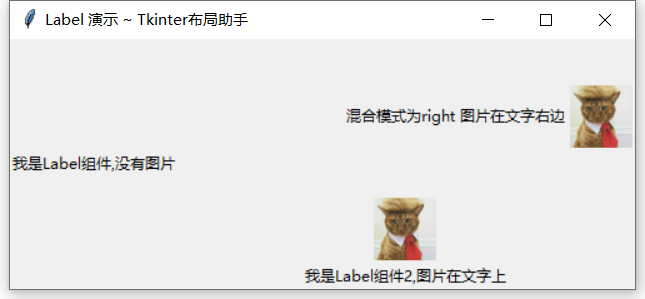
6. Entry 输入框
Entry 组件是 Tkinter 中最常用的图形组件之一,用于接收用户的输入,实现程序与用户的交互。常用于一些表单、数据录入等情景。
常用属性
属性名 | 说明 |
---|---|
background | 背景色 |
foreground | 前景色 文字颜色 |
justify | 文本的对齐方式。取值范围 left (左)、center (中)、right (右)。 |
width | 输入框宽度 |
textvariable | 指定一个变量,设置展示的文本,并在变量变化时,界面展示的文本自动更新 |
show | 当用作密码框时,show="*" |
常用方法
方法名 | 说明 |
---|---|
delete() | 删除内容,传入要删除文字的起始下标 |
get() | 获取输入框的值 |
insert() | 在文本框中插入指定文本 |
数据验证
要开启数据验证,需要设置以下三个选项。
属性 | 说明 |
---|---|
validate | 触发验证的方式。 |
validatecommand | 绑定验证方法 |
invalidcommand | 验证失败的处理 |
validate 取值说明
- focus:获得或者失去焦点的时候验证。
- focusin:获得焦点的时候验证。
- focusout:失去焦点的时候验证。
- key:当输入框被编辑的时候验证。
- all:以上情况都验证。
- none:关闭验证。默认值。
validatecommand 选项需要绑定一个验证方法,该方法只返回布尔值,代表验证是否通过。
invalidcommand 选项用于处理验证未通过的情况,只有在 validatecommand 绑定的方法返回 False 才执行。
Entry 组件示例
1 |
|
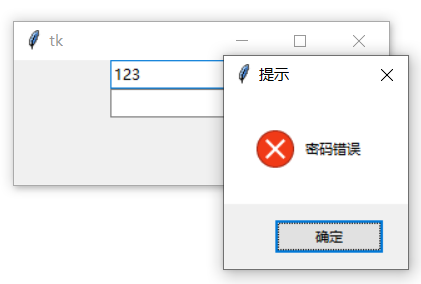
7. Button 按钮
Button 组件是 tkinter 中最常用的组件之一,通常用于绑定(函数)某一操作,用户点击按钮后就会执行该操作。当然按钮也可以不绑定函数,但点击后不会执行任何操作,仅充当展示作用。
常用属性
属性名 | 说明 |
---|---|
image | 背景图片 (建议使用 PIL 的 Image, ImageTk 模块导入图片) |
command | 绑定操作,回调函数,点击按钮时执行 |
state | 按钮状态。 DISABLED (禁用),ACTIVE (激活),NORMAL (默认) |
text | 按钮上的文字 |
textvariable | 指定一个变量,设置展示的文本,并在变量变化时,按钮上的文本自动更新 |
padding | 内边距 (像素) |
Button 按钮组件示例代码
基础使用
1 |
|
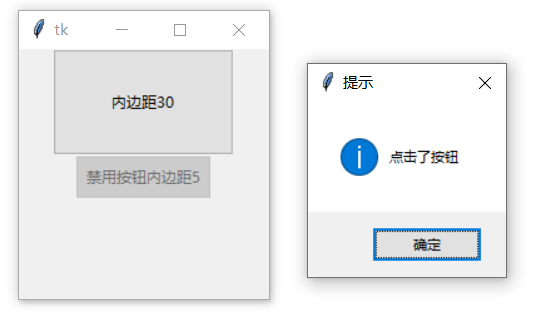
背景图片
1 |
|
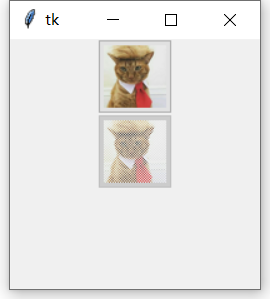
8. Text 文本框
Text 文本框组件是 tkinter 中最常用的组件之一,是比较复杂的组件,一般用于展示多行文本。单行文本通常使用 Entry 输入框组件
Text 文本框组件类型网页中的富文本编辑框,不仅可以编辑文字,还可以设置文字颜色,插入超链接等。Text 文本框组件是 tk 中的组件,在 ttk 中没有对其重新实现。
常用属性
属性名 | 说明 |
---|---|
background | 背景颜色 |
fg | 前景色 文字的颜色 |
bd | 组件边框宽度。默认是 2 像素。 |
selectbackground | 选择文字时的背景色 |
xscrollcommand | 设置水平滚动条 |
yscrollcommand | 设置垂直滚动条 |
insertbackground | 输入框内光标的颜色 |
insertofftime | 光标闪烁时 消失持续时间 默认 300 |
insertontime | 光标闪烁时 显示持续时间 默认 600 |
insertwidth | 光标宽度 默认 2 像素 |
spacing1 | 每个段落的行高 默认 0 |
spacing2 | 一个段落内的行高 (没有回车,超过行宽换行的情况) 默认 0 |
spacing3 | 段落底部,如果有换行在最后一行添 默认 0 |
state | 文本框状态,默认 NORMAL DISABLED (禁用) |
常用方法
属性名 | 说明 |
---|---|
delete(startindex, [,endindex]) | 删除范围内字符,或删除指定字符 |
get(startindex, [,endindex]) | 获取文本内容,获取范围内的文本内容 |
insert(index, [,string]) | 在指定位置插入文本 |
1 |
|
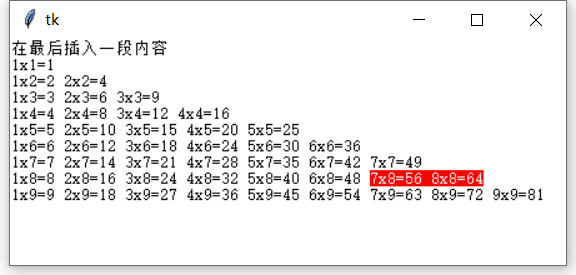
文本框标签相关方法
属性名 | 说明 |
---|---|
tag_add(tagName, index1,index2) | 给指定范围内的文本添加标签 |
tag_bind(tagName,sequence,func) | 给标签绑定事件 |
tag_unbind(tagName,sequence) | 取消标签绑定的事件 |
tag_configure(tagName) | 标签选项配置 |
tag_delete(tagNames) | 删除标签 |
1 |
|
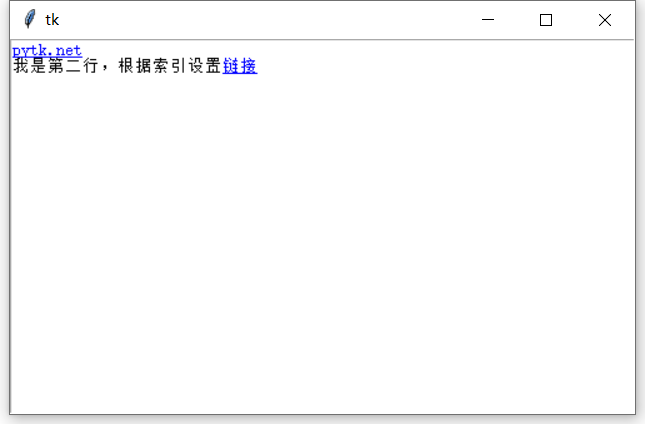
嵌入图片和组件
这个功能还是挺强大的,可以在文本框内嵌入图片和组件,但是能用到的场景比较少。
属性名 | 说明 |
---|---|
image_create | 在文本框嵌入图片 |
window_create | 在文本框嵌入组件 |
1 |
|
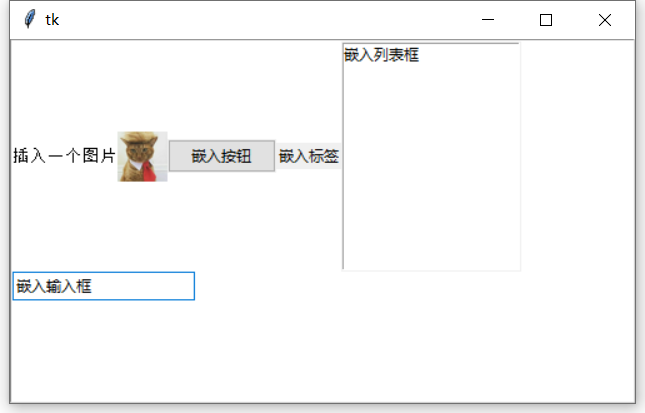
9. Progressbar 进度条
Tkinter 的 Progressbar 进度组件,是 ttk 新增的组件之一,主要用于进度的展示,让用户可以直观的了解到程序的实时进度情况。
进度条主要有两种模式,一种是,指针从起点到终点,用于程序知道当前的进度或完成时间,这也是默认模式。另一种,指针会在起点和终点来回移动,用于程序不确定当前进度或完成时间。
常用属性
属性名 | 说明 |
---|---|
length | 进度条长度 |
maximum | 进度条的最大值 默认 100 |
mode | 模式 determinate (默认) indeterminate (来回移动) |
orient | 进度条方向 默认水平 (horizontal) 垂直 (vertical) |
value | 进度条值 |
variable | 通过变量设置进度条值 取值 IntVar类型 |
常用方法
方法名 | 说明 |
---|---|
start(interval) | 开始展示进度。传参设置隔多久执行一次 step 方法 默认是 50ms |
step(amount) | 进度条数量增加量 默认为 1 |
stop(amount) | 停止进度条 |
进度条组件示例代码
1 |
|
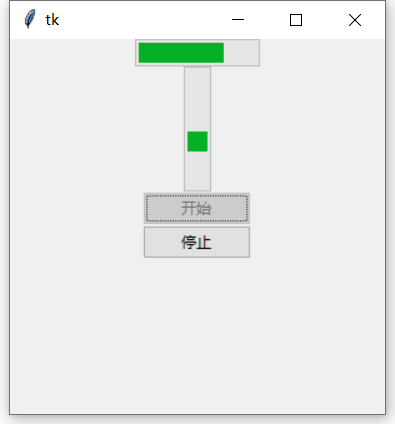
10. Radiobutton 单选框
Radiobutton 单选框,在多个选项下,用户只能选择其中一项。
常用属性
属性名 | 说明 |
---|---|
text | 单选框文字 |
variable | 绑定单选框状态的变量,单选框选择变化后,会将单选框中 value 属性设置的值更新到绑定的变量. IntVar/StringVar 类型 |
单选框示例代码
1 |
|
单选框示例截图
选中单选框后,点击按钮,效果如下。
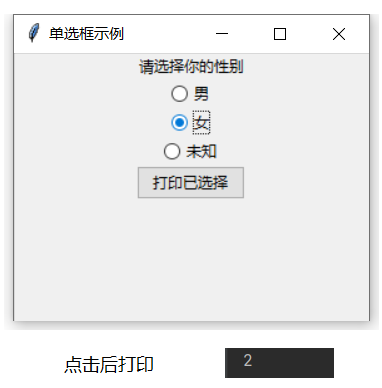